Preprocessor Directives in c
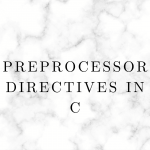
Preprocessor Directives in c, pre-processor instruction call as ‘directives’, directives always start with ‘#’
- Macro replacement
- File Inclusion
- Conditional Compilation
- Replace Comments with empty space
1. Macro:
Macros are symbolic names give to a constant. At the stage of Preprocessor, macros replace with their definitions. There are two types of Macros.
Syntax: #define MACRO_NAME Definition
1. object-like macros: The object-like macros look like a constant. They can’t take arguments.
#define NUM 10
2. function-like macros: Function like same as object-like macros but it can take arguments.
#define SET_BIT(a, p) (a | (1<<p))
Macro Definitions end with newline char. If you want to continue the macro definition with a new line we can use this one ‘\’ end of the line. For Example
#define PRINT_BITS(no, size_bits) {\
int i;\
for(i=(size_bits * 8)-1;i>=0;i--)\
printf("%d ",(no>>i) & 0x01);\
printf("\n");\
}
2. File Inclusion:
File inclusion makes it easy to handle collections of #defines and declarations (among other things). Any source line of the form
#include “filename” or (c pre-processor will look for the specified file in the current directory)
#include<filename> (c pre-processor will look for the specified file in fixed locations)
3. Conditional Compilation:
It is possible to control pre-processing itself with conditional statements that evaluate during pre-processing. This provides a way to include code selectively, depending on the value of conditions evaluated during compilation.
The preprocessor has provided a set of conditional directives which can use to implement conditional compilation.
#ifdef: #ifdef is return true if MACRO_NAME is define, return false if not defined.
Syntax: #ifdef MACRO_NAME
body;
#else
body;
#include <stdio.h>
#define INPUT 1
int main()
{
#ifdef INPUT
int i;
i=2;
printf("%d\n",i);
#else
int i;
printf("Enter a value\n");
scanf("%d",&i);
printf("%d\n",i);
#endif
}
#ifndef: #ifndef is return true if MACRO_NAME is not define, return false if defined.
Syntax: #ifndef MACRO_NAME
body;
#else
body;
#include <stdio.h>
int main()
{
#ifdef INPUT
int i;
i=2;
printf("%d\n",i);
#else
int i;
printf("Enter a value\n");
scanf("%d",&i);
printf("%d\n",i);
#endif
}
#if: #if is return true if the given expression returns a non-zero value, return false if the given expression returns zero.
Syntax: #if EXPRESSION
body;
#else
body;
#include <stdio.h>
int main()
{
#if 1
int i;
i=2;
printf("%d\n",i);
#else
int i;
printf("Enter a value\n");
scanf("%d",&i);
printf("%d\n",i);
#endif
}
Preprocessor Directives in c, “defined” is a unary operator which can use with #if to achieve the same behavior as #ifdef. “!defined” is a unary operator which can use with #if to achieve the same behavior as #ifndef.
Syntax: #ifdef MACRO1
body;
#elif defined MACRO2 /* returns true if macro is defined */
body;
#elif MACRO3 /* returns true if expression returns true */
body;
#elif !defined MACRO4 /* returns true if macro is not defined */
body;
#endif
Operators:
# is a unary operator, # operator will return the given operand enclosed in ” “
## operator will concatenate given operands and returns the concatenated value.
#include<stdio.h>
#define VAL(x,y) x##y
int main(){
printf("%d\n",VAL(10,30));
return 0;
}
Recent Comments