Pointers in C
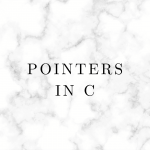
Pointers in C, It is a variable that contains the address of a variable.
Pointer Declaration:
typ_spec *Name;
int a = 20;
int *p; // p is a pointer to integer
p = &a;
pointer size is always equal to the word size of architecture.
on 32-bit – 4 bytes
0n 64-bit – 8 bytes
#include<stdio.h>
int main() {
int *i;
char *c;
double *d;
float *f;
long long int *lli;
printf(" %lu ",sizeof(i));
printf(" %lu ",sizeof(c));
printf(" %lu ",sizeof(d));
printf(" %lu ",sizeof(f));
printf(" %lu\n",sizeof(lli));
return 0;
}
Pointers in C, It should initialize with the return value of the “&” operator or with NULL
Pointer support read/write operations over data with a unary operator “*” called as dereference operator
int *p = NULL;
int a = 10, b;
int *q = &a;
*q=100; /* Write operation on integer data */
b=*q; /* Read operation on data with pointer */
Pointer supports below arithmetic operators
+,- (Binary operators)
++,– (Unary operators)
Increment / Decrement operation with pointers will increment / decrement by data size.
int *p;
p = p+1; // increments by 4 bytes (data size is 4 bytes)
char *c;
c = c+2; // increments by 2 bytes (data size is 1 byte)
Generic or Void Pointers:
Generic pointers are those pointers that can use to store the address of any type of symbol and declaring
void *p;
void pointers can’t be dereference as those pointers consider as pointers to no type.
Those pointers can dereference, first typecast those void pointers to some specific type, and dereference
#include <stdio.h>
int main()
{
int a = 10;
void *p;
p = &a;
*(int *)p = 100;
printf("%d %d", a, *(int *)p);
return 0;
}
Pointer to const :
The pointer to constant variable can’t dereference for the write operation. Formally syntax is
const data_type *name;
const int *p; // pointer to integer constant
Constant pointers:
The Constant pointer variable can be a dereference for reading and write operation, can’t update with the new address. Formally syntax is
data_type *const name;
int *const p;
Constant pointer to constant:
The Constant pointer to constant variable can’t dereference read and write operation, can’t update with the new address. Formally syntax is
const data_type *const name;
const int *const p;
Recent Comments