Linux System programming interview question and Answers
Linux System programming interview question and Answers
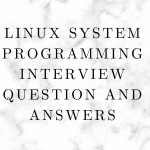
1. What is the use of system command?
Ans: The System() command is used to execute a shell command.
2. How to switch and access user mode to kernel mode?
Ans: user application can’t access kernel space directly and similarly kernel code can’t access the userspace. user and kernel space can communicate with each other using various ways.
ioctl, procfs, sysfs, NetLink sockets.
3. Difference between fork, vfork, and clone?
Ans: fork, vfork, and clone are used to create new child processes. fork make a copy of address space and vfork does not make a copy of address space. In fork the first parent will execute then the child will execute. if you want to execute child first then use wait() call in the parent code block. the vfork function executes the child process first then the parent will execute.
clone function creates a new child process that shares memory, file descriptors, and signal handlers with the parent. the child terminates when the parent terminates.
4. What is the zombie process?
Ans: A zombie process is a process whose execution is completed but it still has an entry in the process table. Zombie processes usually occur for child processes, as the parent process still needs to read its child’s exit status. Once this is done using the wait system call, the zombie process is eliminated from the process table. This is known as reaping the zombie process.
5. Difference between Process and thread?
Ans: A program in execution is often referred to as a process. a thread is a subset of the process.
the process has its own address space. thread uses the process address space and shares it with the other threads of that process.
a thread can communicate with other threads directly by using methods like wait(), notify(). a process can communicate with another process by using inter-process communication.
thread is easy to create and maintain. process are difficult to create and maintain.
6. Difference between the static library and dynamic library?
Ans:
Static:
They contain fully resolved library functions that are physically linked to the executable images during building.
Dynamic:
They contain symbolic references to library functions used and these references are fully resolved either during application load time or run time.
7. How to create static and dynamic libraries?
Ans: Executables can be created in two ways
1. Static: They contain fully resolved library functions that are physically linked into the executable images during built.
creating static libraries:
1. Implement library source files.
2. compile and generate relocatable files.
gcc -c example.c -o example.o
3. use ar tool for creating a static library using all those relocatable files created it step 2
ar -rcs libexample.a example.o
To compile gcc main.c ./libexample.a -o mainstat
4. all libraries in Linux start with ‘lib’ followed by name and extension is .a they are considered static libraries.
2. Dynamic: They contain symbolic references to library functions used and these references are fully resolved either during application load time or run time.
creating dynamic libraries:
1. Implement library source files.
2. compile and generate position Independent relocatable files.
gcc -c -fPIC example.c -o example.o
3. invoke gcc with -shared flag for creating a shared object.
gcc -shared example.o -o libexample.so
To compile gcc main.c ./libexample.so -o maindyn
4. all libraries in Linux start with ‘lib’ followed by name and extension is .so they are considered as dynamic libraries.
In both, the cases output will be the same. In order to understand the major differences between static and dynamic linking, we will analyze the executables using objdump tool.
objdump -D maindyn
8983845: ca 00 00 00 00 call 0892340 <example@plt>
Here in dynamic executable’s main function, it is calling example@plt , (plt= procedure linkage table). Plt table is generated by the linker and contains information about dynamic linking.
8.What is the difference between fopen and open?
Ans: fopen is c standard library function for handling the opening of the files as stream objects. open is a system call for handling the opening of the files as file descriptors.
9. Difference between semaphore and mutex?
Ans: Mutex uses a locking mechanism i.e. if a process wants to use a resource then it locks the resource, uses it, and then releases it. But on the other hand, semaphore uses a signaling mechanism where wait() and signal() methods are used to show if a process is releasing a resource or taking a resource.
In mutex, the lock can be acquired and released by the same process at a time. But the value of the semaphore variable can be modified by any process that needs some resource but only one process can change the value at a time
10. What is the sequence (socket API) to create a simple listening server?
Ans: socket()
bind()
listen()
accept()
recevfrom()
sendto()
11. What is interrupt and how to create intrepputs in linux?
Ans: Linux kernel configures do_irq() routine as a default response function for all external interrupts. do_irq() is the routine of process 0, which is responsible for allocation of interrupt stack and invoking appropriate interrupt service function.
12 What is cgroups?
Ans: Control groups, usually referred to as cgroups, are a Linux kernel feature that allow processes to be organized into hierarchical groups whose usage of various types of resources can then be limited and monitored.
The kernel’s cgroup interface is provided through a pseudo-filesystem called cgroupfs. Grouping is implemented in the core cgroup kernel code, while resource tracking and limits are implemented in a set of per-resource-type subsystems (memory, CPU, and so on).
13. How to create a child process in the kernel?
Ans: fork, vfork, clone
14. What is the IPC mechanism and different types of IPC’s?
Ans: Signals, Sockets, Synchronization, message queues, shared memory.
15. What is signal and write own signal handler?
Ans: A system message sent one from one process to other, not usually used to transfer data but instead used to remotely command the partnered process.
void sig_handler(int sig) {
printf(“Enter to own signal handler\n”);
}
signal(SIG_INT, sig_handler);
16. What is the difference between message queues and shared memory? who is faster?
Ans: The message-passing model allows multiple processes to read and write data to the message queue without being connected to each other. Messages are stored on the queue until their recipient retrieves them. Message queues are quite useful for interprocess communication and are used by most operating systems.
However, the message passing model has slower communication than the shared memory model because the connection setup takes time.
The shared memory in the shared memory model is the memory that can simultaneously access by multiple processes. This is done so that the processes can communicate with each other. All POSIX systems, as well as Windows operating systems, use shared memory.
An advantage of the shared memory model is that memory communication is faster as compared to the message-passing model on the same machine.
However, the shared memory model may create problems such as synchronization and memory protection that need to be addressed.
17. What are the synchronization mechanisms?
Ans: there are two kinds of locking primitives provide by the thread libraries.
1) Wait locks
a. Mutex
b. semaphore
2) Poll locks.
a. Spin locks
The wait locking interface pushes the caller process into a wait state when lock acquisition fails. These recommend when critical sessions are long and undeterministic. Poll locking interface puts the caller process into a loop until the lock is available. These recommend when the critical sessions are short and deterministic.
18. Can we use mutex objects outside of the process?
Ans: no, From the term mutual exclusion, we can understand that only one process at a time can access the given resource. The mutex object allows the multiple program threads to use the same resource but one at a time not simultaneously.
19. Can we use semaphore outside of the process?
Ans: yes, process synchronization
Semaphore variable value can be modified by any process that acquires or releases resources by performing wait() and signal() operations.
20. What is vfs and the use of vfs?
Ans: The Virtual File System (also known as the Virtual Filesystem Switch) is the software layer in the kernel that provides the filesystem interface to userspace programs. It also provides an abstraction within the kernel that allows different filesystem implementations to coexist.
VFS system calls open(2), stat(2), read(2), write(2), chmod(2) and so on calls from a process context.
21. What is interrupt latency?
Ans: Interrupt latency is about the total amount of time spent by a system to respond an interrupt.
1. hardware latency
2. kernel latency
3. interrupt handler latency
4. soft interrupt latency
5. scheduler latency
22. What are top and bottom halves?
Ans: Top halves: it is executing as an immediate response to interrupt.
bottom halves: it is executing some time later when cpu gets free time.
23. How kernel memory allocates using kmalloc and kfree?
Ans: kmalloc and kfree are slab allocators. it will allocate block memory.
24. What is memory management?
Ans: The memory management subsystem is one of the most important parts of the operating system. Allocate a page list with an instance of struct page representing each physical frame. categorize pages into appropriate zone.
1. zone DMA (0 – 16 MB)
2. zone normal (16 – 896 MB)
3. zone high (>896 MB)
25. What is the POSIX thread?
Ans: Pthreads are user-level threading libraries for UNIX and its variants.
26. Can we run multiple threads in parallel?
Ans: yes, we can run multiple threads parallel.
27. What are the attributes of POSIX thread?
Ans: thread attributes 1. Detach state 2. scope 3. stack 4. scheduling
28. What is scheduling and how can schedule multiple threads?
Ans: Defines scheduling policy and priority. Linux kernel supports the following policies by default.
non-real-time — SCHED_OTHER, SCHED_BATCH, SCHED_IDLE
realtime —- SCHED_RR, SCHED_FIFO
29. What is context switching?
Ans: context switch is the process of storing the state of a process or thread so that it can be restored and resume executing at a later point. this allows multiple processes to share a single central processing unit and is an essential feature of a multitasking operating system.
30. What is a process control block?
Ans: every process has an address space in user mode and PCB in kernel mode.PCB stores each process information. elements in PCB task_struct, mm_struct, tty_struct, file_struct, signal_struct.
Recent Comments