File I/O Operations in Linux
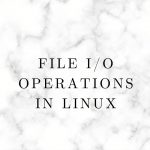
File I/O Operations in Linux
open:
#include <sys/stat.h>
#include <fcntl.h>
int open(const char *pathname, int flags);
int open(const char *pathname, int flags, mode_t mode);
The open() system call opens the file specified by pathname. If the specified file does not exist, it may optionally (if O_CREAT is specified in flags) be created by open().
The argument flags must include one of the following access modes: O_RDONLY, O_WRONLY, or O_RDWR. These request opening the file read-only, write-only, or read/write, respectively.
optional flags can be passes by using bitwise operators
O_APPEND – file is opens in append mode
O_CREAT – If the specified file does not exist it will be created
O_TRUNC – If the file is already existing then the file size is truncates to zero
mode specifies the permissions to uses in case a new file creates. this argument is valid if and only if the flag argument includes O_CREAT.
On success, open() return the new file descriptor (a nonnegative integer). On error, -1 returns, and errno sets to indicate the error.
close:
#include <unistd.h>
int close(int fd);
close() closes a file descriptor, so that it no longer refers to any file and may be reused.
close() returns zero on success. On error, -1 returns, and errno sets to indicate the error.
write:
#include <unistd.h>
ssize_t write(int fd, const void *buf, size_t count);
write() writes up to count bytes from the buffer starting at buf to the file referred to by the file descriptor fd.
On success, returns the number of bytes written. On error, -1 returns, and errno sets to indicate the error.
read:
#include <unistd.h>
ssize_t read(int fd, void *buf, size_t count);
read() attempts to read up to count bytes from file descriptor fd into the buffer starting at buf.
If the count is zero, read() returns zero and has no other results. if the count is greater than SSIZE_MAX, the result is implementation-defined.
on success, returns the number of bytes read. on error, -1 returns and errno sets appropriately.
lseek:
#include <unistd.h>
off_t lseek(int fd, off_t offset, int whence);
lseek() repositions the file offset of the open file description associated with the file descriptor fd to the argument offset according to the directive whence as follows:
SEEK_SET – The file offset sets to offset bytes.
SEEK_CUR – The file offset sets to its current location plus offset bytes.
SEEK_END – The file offset sets to the size of the file plus offset bytes.
Upon successful completion, lseek() returns the resulting offset location as measured in bytes from the beginning of the file. On error, the value (off_t) -1 returns and errno sets to indicate the error.
#include<stdlib.h>
#include<sys/types.h>
#include<sys/stat.h>
#include<fcntl.h>
#include<unistd.h>
#include<string.h>
struct student{
char name[25];
int no;
char gen;
};
int main() {
int fd, ret, i;
struct student s[3] = {{"satheesh",1,'m'},{"kumar",2,'m'},{"nelavalli",3,'m'}};
struct student rs[3];
fd = open("file.txt",O_RDWR|O_CREAT|O_TRUNC,0666);
if(fd < 0) {
perror("open");
return -1;
}
ret = write(fd,s, sizeof(s));
if(ret < 0) {
perror("write");
return -1;
}
lseek(fd, 0, SEEK_SET);
ret = read(fd, rs, sizeof(rs));
if(ret < 0) {
perror("read");
return -1;
}
for(i=0; i<3;i++) {
printf("%s %d %c \n", rs[i].name, rs[i].no,rs[i].gen);
}
close(fd);
return 0;
}
Recent Comments